First, you have to install a fresh version of Laravel 10. You can go to the official documentation of Laravel:
You will see a section there: "Creating A Laravel Project". Here you will get your codes to install Laravel 10. I am going to write the commands you will need to install laravel. First, open your terminal where you want to install laravel on your computer. Then use these commands:
composer create-project laravel/laravel example-app
cd example-app
php artisan serve
You can run your application now using this url into your browser: http://127.0.0.1:8000
Now go to this location: resources > views > welcome.blade.php.
Change the codes of this page like this:
Change the codes of this page like this:
welcome.blade.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Laravel - Stripe Integration</title>
</head>
<body>
<h2>Product: Laptop</h2>
<h3>Price: $5</h3>
<form action="{{ route('stripe') }}" method="post">
@csrf
<input type="hidden" name="price" value="5">
<input type="hidden" name="product_name" value="Laptop">
<input type="hidden" name="quantity" value="1">
<button type="submit">Pay with Stripe</button>
</form>
</body>
</html>
I have these information according to the last episode:
Email Address: xisic28605@ikanid.com
Publishable key: pk_test_51OFZ3zI3hY1Jc4DzUtimeKqiANmmSvju1Rtkz2HxqY0pySZx3fVkpGUyB0r8w0OBI5jejmJPjoVGgrLhrO3tItnG00OhIjk4Dw
Secret key: sk_test_51OFZ3zI3hY1Jc4Dzx4VMcmJkeEVqrXuL1ShIv5RgH7FD7JCeHiO8wUuKbwDfV2h2nZ36NcRT3oSHxvrKCZbs1Qzu00clvUfgOY
Go to the .env file and add these lines in the bottom:
STRIPE_TEST_PK=pk_test_51OFZ3zI3hY1Jc4DzUtimeKqiANmmSvju1Rtkz2HxqY0pySZx3fVkpGUyB0r8w0OBI5jejmJPjoVGgrLhrO3tItnG00OhIjk4Dw
STRIPE_TEST_SK=sk_test_51OFZ3zI3hY1Jc4Dzx4VMcmJkeEVqrXuL1ShIv5RgH7FD7JCeHiO8wUuKbwDfV2h2nZ36NcRT3oSHxvrKCZbs1Qzu00clvUfgOY
Go to the Stripe documentation from here:
You will get a search box in the top. Search there by "Quantity Checkout". Click on the top link that comes here.
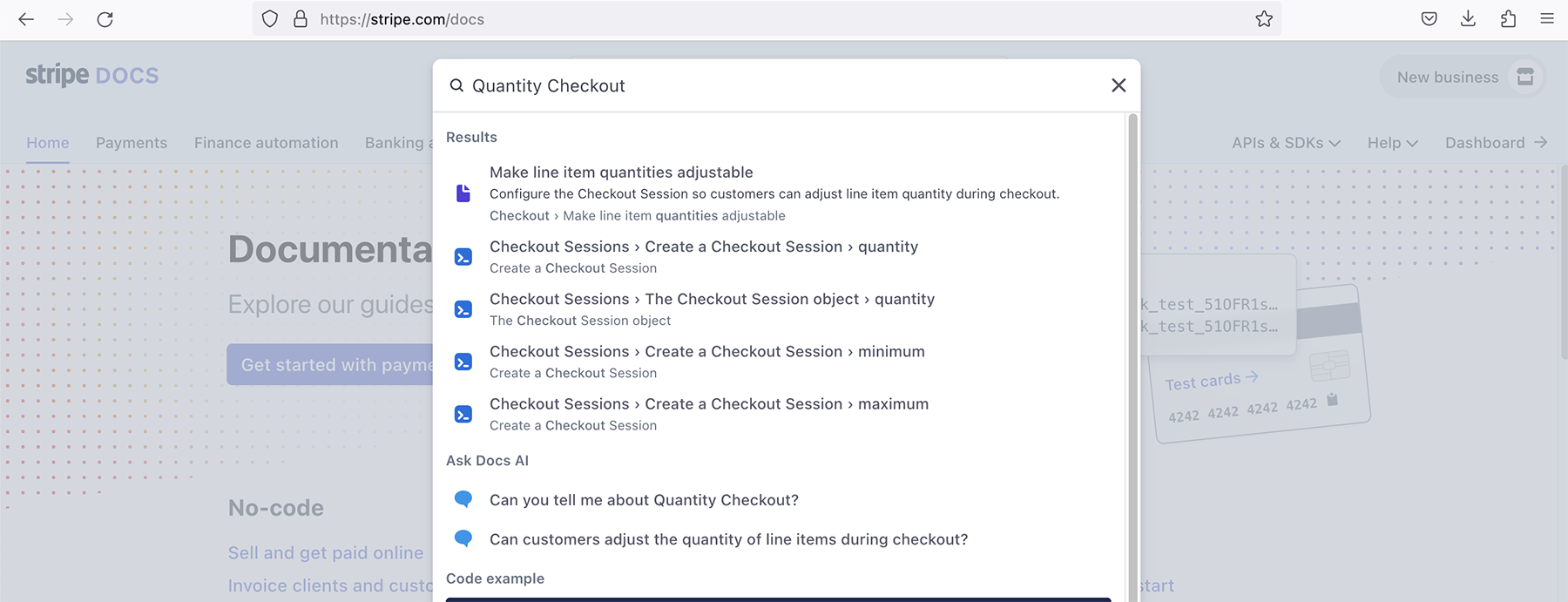
Go to the bottom and you will get a code section. From there select php and copy all the codes.
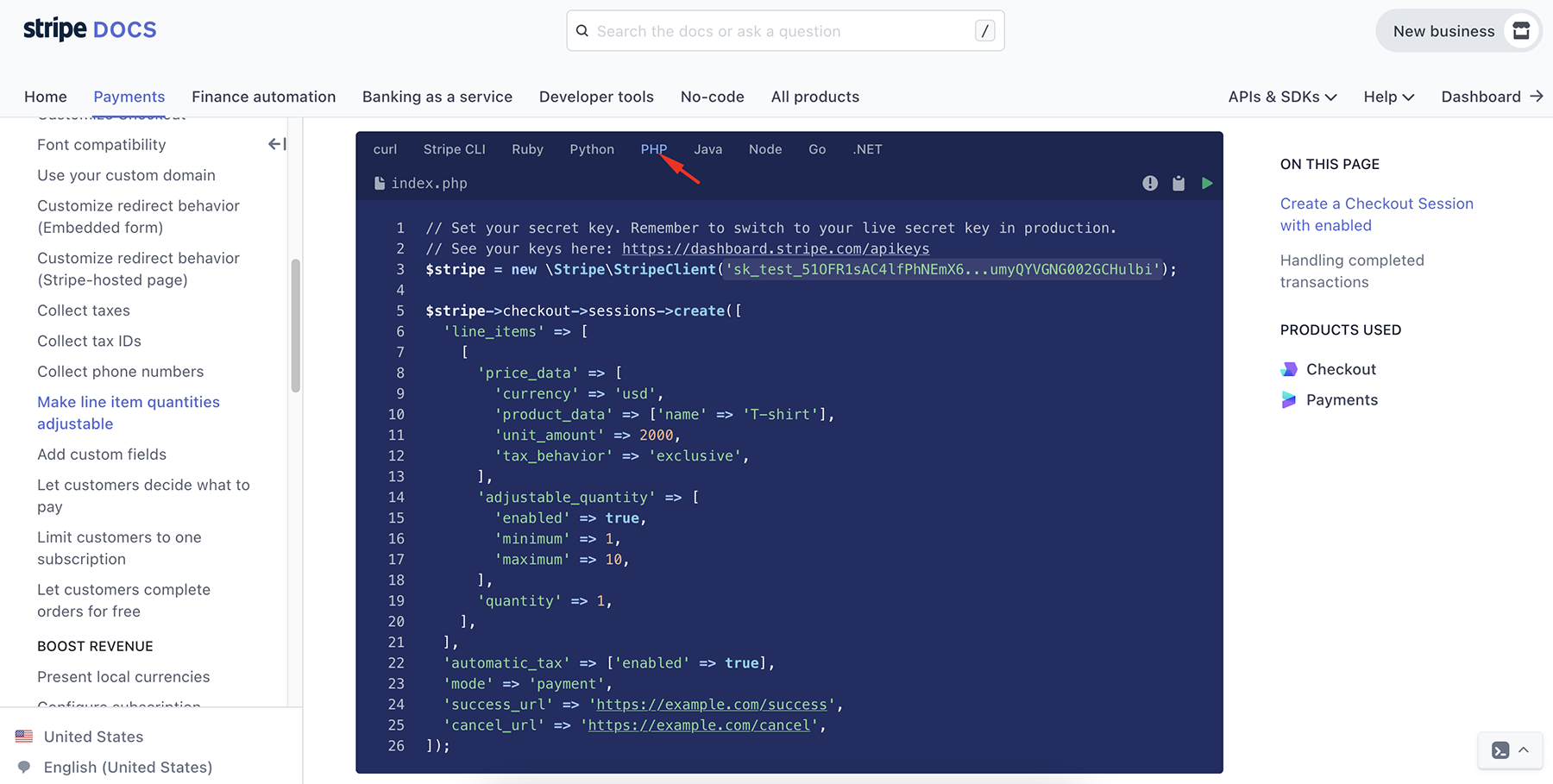
You can now use this code into your controller.
// Set your secret key. Remember to switch to your live secret key in production.
// See your keys here: https://dashboard.stripe.com/apikeys
$stripe = new \Stripe\StripeClient('sk_test_51OFR1sAC4lfPhNEmX6zAtr20XXzKaonx6PSrT5jrfOVnNKGLK8JKYIgRj8sUiwsIEFoo6iBzv9SpwgnumyQYVGNG002GCHulbi');
$stripe->checkout->sessions->create([
'line_items' => [
[
'price_data' => [
'currency' => 'usd',
'product_data' => ['name' => 'T-shirt'],
'unit_amount' => 2000,
'tax_behavior' => 'exclusive',
],
'adjustable_quantity' => [
'enabled' => true,
'minimum' => 1,
'maximum' => 10,
],
'quantity' => 1,
],
],
'automatic_tax' => ['enabled' => true],
'mode' => 'payment',
'success_url' => 'https://example.com/success',
'cancel_url' => 'https://example.com/cancel',
]);
In .env file, setup database name, username and password. In my case, it is:
DB_DATABASE=stripe
DB_USERNAME=root
DB_PASSWORD=root
In config folder, create a file: "stripe.php". Write the following codes there:
<?php
return [
'stripe_pk' => env('STRIPE_TEST_PK'),
'stripe_sk' => env('STRIPE_TEST_SK'),
];
There are many packages available using which you can integrate stripe easily in your application. I am going to use this for this application:
Now install the package using this command:
composer require stripe/stripe-php
Create a migration file using this command:
php artisan make:migration create_payments_table
A migration file will be created in this location: "database > migrations > 2023_11_23_093629_create_payments_table.php"
Now write the following code here:
Now write the following code here:
2023_11_23_093629_create_payments_table
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('payments', function (Blueprint $table) {
$table->id();
$table->string('payment_id');
$table->string('product_name');
$table->string('quantity');
$table->string('amount');
$table->string('currency');
$table->string('customer_name');
$table->string('customer_email');
$table->string('payment_status');
$table->string('payment_method');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('payments');
}
};
Write this command to create the "payments" table.
php artisan migrate
You have to create a model now. The model name will be the singular form of the table name. So it will be "Payment". Run this command in the terminal to create this model:
php artisan make:model Payment
I am going to create a controller now. So run this command in terminal:
php artisan make:controller StripeController
StripeController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Payment;
class StripeController extends Controller
{
public function stripe(Request $request)
{
$stripe = new \Stripe\StripeClient(config('stripe.stripe_sk'));
$response = $stripe->checkout->sessions->create([
'line_items' => [
[
'price_data' => [
'currency' => 'usd',
'product_data' => [
'name' => $request->product_name,
],
'unit_amount' => $request->price*100,
],
'quantity' => $request->quantity,
],
],
'mode' => 'payment',
'success_url' => route('success').'?session_id={CHECKOUT_SESSION_ID}',
'cancel_url' => route('cancel'),
]);
//dd($response);
if(isset($response->id) && $response->id != ''){
session()->put('product_name', $request->product_name);
session()->put('quantity', $request->quantity);
session()->put('price', $request->price);
return redirect($response->url);
} else {
return redirect()->route('cancel');
}
}
public function success(Request $request)
{
if(isset($request->session_id)) {
$stripe = new \Stripe\StripeClient(config('stripe.stripe_sk'));
$response = $stripe->checkout->sessions->retrieve($request->session_id);
//dd($response);
$payment = new Payment();
$payment->payment_id = $response->id;
$payment->product_name = session()->get('product_name');
$payment->quantity = session()->get('quantity');
$payment->amount = session()->get('price');
$payment->currency = $response->currency;
$payment->customer_name = $response->customer_details->name;
$payment->customer_email = $response->customer_details->email;
$payment->payment_status = $response->status;
$payment->payment_method = "Stripe";
$payment->save();
return "Payment is successful";
session()->forget('product_name');
session()->forget('quantity');
session()->forget('price');
} else {
return redirect()->route('cancel');
}
}
public function cancel()
{
return "Payment is canceled.";
}
}
Now go to "route > web.php"
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\StripeController;
Route::get('/', function () {
return view('welcome');
});
Route::post('stripe', [StripeController::class, 'stripe'])->name('stripe');
Route::get('success', [StripeController::class, 'success'])->name('success');
Route::get('cancel', [StripeController::class, 'cancel'])->name('cancel');
See Full Tutorial on YouTube
If you want to see the full functionality in live, you can see my video on youtube.
Download Source Code
Please give your email address. We will send you the source code on your email.